Send & Receive Input Messages
Inputs allow a Twin to receive specific messages from any authorised Twin in the Network.
Introduction to Input Messages
By advertising an Input, an IOTICS Digital Twin allows authorised Twins to send direct messages to it. This way, Inputs can be used to send commands to change the state of the underlying asset or to send messages that can be interpreted by the Twin to implement a request/response way of interaction.
Feeds vs. Inputs
- Inputs are similar to Feeds, they both possess properties and values to describe how the underlying data, which will be transferred, is expected to look.
- Compared to a Feed, which allows a Twin to share data to any authorised Twin in the Network (one-to-many communications), an Input consents any authorised Twin to share data to a specific Twin (many-to-one communications).
- Feeds are generally used to continuously import data into an IOTICSpace that can be potentially followed by any Twin in the Network (i.e.: temperature data, weather forecast update, etc.). Inputs are useful to send event-based messages to specific Twins so the latter can respond accordingly (i.e.: client/server communication) or modify the behaviour of the physical asset (i.e.: switch a light on/off).
- Selective Data Sharing applies to both Feeds and Inputs by using the same AllowList property.
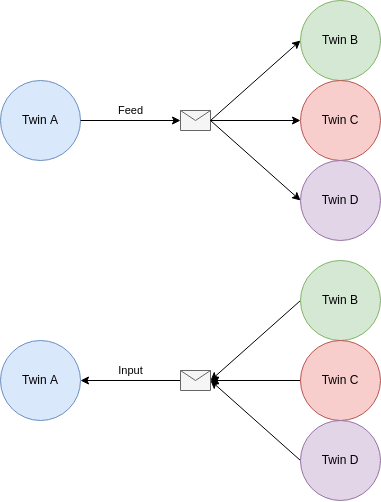
Feeds vs. Inputs
Need a refresher on Twin Feeds?
Our previous guide Share Data & Follow Feeds can help you!
Create an Input
A Twin can have none, one or many Inputs as well as a combination of Inputs and Feeds.
How to create an Input with the IOTICS API
An Input can be created through the use of the Create Input operation. Its structure is composed of the same components as Feeds:
- Basic Structure: it includes Input ID only;
- Metadata: made up of a list of Properties;
- Values: the actual payload included in the Input message which is divided into Label, Comment, Datatype and Unit.
Be aware that, like the Create Feed operation, Create Input allows to create only the Basic Structure of the Input. In order to add the remaining components (Metadata and Values) the Update Input operation must be used.
Send and Receive Input Messages
In order for a Twin A (sender) to send an Input message to another Twin B (receiver), Twin A needs to be authorised by Twin B in terms of both:
- Visibility: Twin B needs to be found from the IOTICSpace where Twin A lives;
- Accessibility: Twin A's IOTICSpace needs to be authorised to share data with Twin B.
To see an example on how to receive Input messages see:
To see an example on how to send Input messages see:
Updated 8 months ago